AudienceData
Adserver
Best Practices
Do's and dont's when using AudienceData
How to document the accuracy?
Why introduce segments with different affinities?
Using targeting with the right conditioning
DSP
How to access data AudienceData segments in Adform
How to find AudienceData in MediaMath DMP
How to find segments in BidTheatre
How to find segments in Display & Video 360
Data Introduction
Available Segments
Existing integrations
Methodology and precision
The distinction between campaign impression profile and impressions in target group
What is deterministic data?
What is probabilistic data?
Publisher
Accessing targeted data with DFP Audience sync
AdForm publisher integration instructions
How to find data usage information in Google 360 for Publishers former DFP
How to report on AudienceProject segment usage in DFP
Inventory available for realtime targeting in DFP
Lotame integration
Sending targeting key values to AdManager
Troubleshooting
AudienceHub
How to create your first audience
How to create your first seed
Case 1: Selecting a customer file
Case 2: Selecting an Amazon S3 file
Case 3: Selecting survey data from UserReport
Creating a seed
Insights reports
What is AudienceHub?
The new generation of AudienceReport
API Documentation
API Best Practices
How do I use the AudienceReport Next API?
Understanding the API documentation
What is an API?
Where do I find the API key?
Account Management
Account Types
Agencies: managing user access for connected accounts
How to add new clients
How to connect an Agency account to a Premium client account and vice versa
How to disconnect accounts
How to manage user accounts
User roles
What is the 2-step verification and how does it work?
Integrations
Adform
Amazon Ads
CTV and Addressable TV
Campaign Manager
DV360
Facebook/Meta
Semi-Automated integrations
TechEdge Integration
YouTube
Measurement Methodology
Pixel Implementation
Getting Started with Pixels
How do URL-Parameters work?
How to add parameters to AudienceReport pixel
How to check if your pixel is firing?
How to create a pixel?
SSL - Compliance
The GDPR parameters
What is a CACHE-Buster and why do we need it?
What is a tracking pixel?
What is the purpose of a t-code?
Setting up Pixels
Pricing
Reports
Creating and Sharing reports
How to add and export tracking pixels to your reporting items
How to add custom report items
How to duplicate a report
How to export your report
How to share your report with your client
How to understand your report
How to understand your report - Dashboard
How to understand your report - Delivery
How to understand your report - Funnel
How to understand your report - Profile
How to understand your report - Reach
How to use an exported pixel
Getting Started with Reports
The original AudienceReport
Addressable TV
Activating Addressable TV measurement
Available Addressable TV device types
How Addressable TV is measured
How to get the Addressable TV measurement tool in AudienceReport
Impact on sample size and frequency
Sharing Addressable TV measurement numbers with TechEdge
What is Addressable TV?
Adserver Implementation
Ad Tech
Adserver - Adform
Adserver - VideoPlaza
Atlas
Double Click DCM Adserver
Emediate
Extented Sizmek Asia-guide
How to implement creative wrapper in Ad Manager
Programmatic Publisher Ad Server - Adform PPAS
Setting-up video measurement in Google Ad Manager
Sizmek Ad Suite Tracking
Sizmek/MediaMind guide
Tracking using JavaScript
Implementing AudienceReport tracking pixels in Webspectators
Brand Lift Studies
Cache-buster
Is my cache-buster working?
What is a cache-buster?
Which cache buster shall I use for my ad server?
Why do we need a cache-buster?
Creating Projects
Adding tracking points / pixels to your project
Applying filters to your data
Change your target group or report period
Creating your first project
Duplicating campaigns
How to merge projects
How your data will change when applying filters
Custom Segments
Activating your Customer Segments 3.0
Available Custom Segments
Custom Segments 3.0
Custom Segments and Sample Size
Reach, Coverage and Segments Availability
What are Custom Segments?
Event Tracking
Adding tracking points / pixels with event tracking to your project
Event tracking in various adservers
Implementing click trackers
In-app tracking
In-view tracking of inlined content
Understanding Event Tracking
What is Event Tracking?
Integrated Report
Connect your Facebook Business Manager account to AudienceReport
Connect your Google Ads account to AudienceReport
Connect your Google Display & Video 360 account to AudienceReport
How are data integrated?
How to create an Integrated Report
To-do list before creating an Integrated Report
Understanding your Integrated Report
What is an Integrated Report?
Integrations
Adform integration set-up
Automatic tracking of DFP campaigns
Google Campaign Manager Integration
Integrate AudienceReport and Techedge AxM (AdvantEdge Cross Media)
Intercept
Pixel implementation
Quality
How Transparency is measured
How Viewability is measured
How the Overall Quality Score is calculated
Viewability tracking using AudienceReport measurement script
What is Quality?
What is a good Quality score?
What is a hidden referrer or a generic referrer?
What is the difference between no referrer and other referrers (in the tracking point violations table)?
When is a tracking point considered to be violating Geo Compliance/Transparency/Viewability?
Why can’t I drill down on some countries to see in which regions my impressions are loaded?
Why is my overall score not that bad when almost all my impressions are of low quality?
Why is there a discrepancy between the impression count in the Quality tab and the rest of the report while my campaign is running?
Will my viewability score of 0.0 affect the overall Quality score if I didn’t implement in-view tracking?
Reports
Customized PDF reports
Deeper Insights with Campaign Audience Measurement
Exporting your report
How to search for your project
Introducing the common affinity profile
Managing your projects with labels
Sample sizes
Tired of clicks and imps?
Understanding your project
Technical Questions
Account Administration
Ad blocking
Can I change the phone number I chose for the two-step verification process?
Checking SSL-Compliance
General Troubleshooting
Getting started with AudienceReport API
How do URL-parameters work?
How often will I be asked to log in through the two-step verification process?
How to track traffic by device type
If you accidentally delete pixels from your project
The procedure to enable the two-step verification
What if I lose my phone and cannot access my account?
Tracking Pixels
Upgrade to the new generation of AudienceReport
AudienceReport Upgrade FAQ
Comparing the original and the new generation of AudienceReport
How to import data from the original AudienceReport
UserReport
Installing UserReport and setting up your media sections
Defining your website in the media section
General Account Information
Installing UserReport on your website or app
Adding own user ID to UserReport
Configure Google Tag Manager to resolve media dynamically
Configuring media/section through snippet
Install UserReport script with Google Tag Manager
Installing UserReport SDK to Android Application
Installing UserReport SDK to iOS application
Installing UserReport script on your website
Kits
General Information
Reach and Coverage of Custom Segments
Target Audience verified by Kits
The technology behind Kits
What are Kits?
Getting started
Troubleshooting
Working with Kits
The feedback widget
Activate the Feedback widget
Adding context information to ideas and bugs
Customize Feedback widget buttons and links
Customize color, text and position of the Feedback widget
Disabling the Feedback widget on specific devices
Get direct link to the Feedback widget or manually display it
How to activate your Feedback widget
How to change the status of an idea or add a comment
How to disable the "report a bug" feature
Is the Feedback Forum available in my language?
Pre-populating username and email
What is the Feedback widget?
The feedback report
The survey widget
Activate Email Collect
Activate Net Promoter Score ®
Activate the Survey widget and create your questions
Chained questions and how they work
Controlling invitation frequency when using UserReport script and Google Tag Manager
How many questions can be added?
How many surveys answers do I need?
How to add questions to your survey
How to customise you survey widget
How to deactivate and delete your survey questions
How to show or hide results from users
Is UserReport 100% free?
Is the UserReport survey widget available in my language?
Managing invitation rules through Ad-server
Preview your survey
Respecting the user
User invitation to UserReport survey and the quarantine system
Who pays for the prizes in the survey?
Will UserReport slow down my website? Is it affected by popup blockers?
The Google Analytics Integration
The survey reports
Accessing Newsletter signups using API
- All Categories
- UserReport
- Installing UserReport and setting up your media sections
- Installing UserReport on your website or app
- Configuring media/section through snippet
Configuring media/section through snippet
General information
We offer convenient tools to resolve Media and Sections from URL, however, there are cases, when it can not be achieved. There are a few examples:
- A website has multiple languages on the same domain, and current language is not reflected in the website address (for instance stored in a cookie)
- A website has a lot of visitors and a lot of different content. For instance big Forum with different categories (from cooking to car-fixing guides) and subcategories in each. Content and audience is different, so each category can be defined as defined
- Website's Content Management System (CMS) allows content categories, however, a category is not present in page address. For instance, the page address is example.com/news/123 and content belong to Sports category, on page example.com/news/456 to Politics
Cases like this require programmatic media setting in UserReport script implementation. There are two ways:
- Using
meta
header displayed on the Advanced tab - Using JavaScript API
The first approach is straightforward and should be implemented on server-side. However more often UserReport is deployed through various Tag managers, like Google Tag Manager, Permutive etc. So here is small API how to set media/section:
<script>
window._urq = window._urq || [];
window._urq.push(["setMediaId", "MEDIA_ID"]);
window._urq.push(["setSectionId", "SECTION_ID"]);
</script>
You can find MEDIA_ID
and SECTION_ID
values in meta-tag snippet shown on the Advanced tab of Media/Section configuration. These methods must be invoked before SAK loaded and executed. Therefore complete snippet should have following look
<script>
window._urq = window._urq || [];
window._urq.push(["setMediaId", "MEDIA_ID"]);
window._urq.push(["setSectionId", "SECTION_ID"]);
</script>
<script src="https://sak.userreport.com/your_company/launcher.js"
id="userreport-launcher-script" async></script>
Warning: pageview is assigned to one media, however, may be associated with multiple sessions. Therefore setMediaId
must be called once, setSectionId
may be called multiple times, so a pageview will count to impressions on these sections.
Setting Media ID
Once you have created you may not specify URL-based rules, obtain media ID and from meta tag shown on the Advanced tab, like on the example below
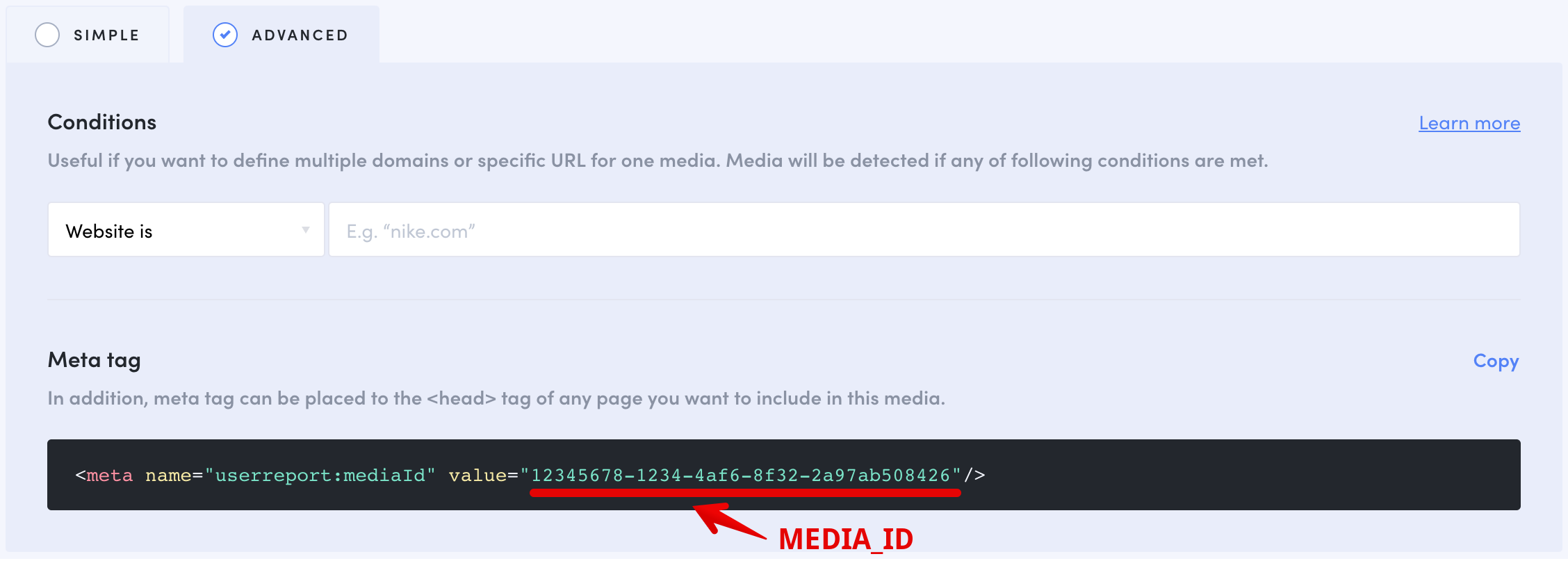
Having this value you can place a snippet
<script>
window._urq = window._urq || [];
window._urq.push(["setMediaId", "12345678-1234-4af6-8f32-2a97ab508426"]);
</script>
<script src="https://sak.userreport.com/your_company/launcher.js"
id="userreport-launcher-script" async></script>
This will run UserReport same survey regardless of a domain where the snippet is deployed.
Example. Choosing media ID based on the cookie value
Your website has multiple languages (English, Danish, and German) on the same domain (example.com) and current language is not reflected in the website address but stored in the cookie with name lang. You need to do following:
- Create three UserReport media for each language, don't enter media detection rules
- Get ID of each created media
- Modify UserReport snippet so it gets language from cookie, chooses correct media and sets it to snippet
Here is example, where website language is stored in cookie with name lang
that has one of possible values - da, en, de
.
<script>
function initUserReport() {
// Relation between language (key) and corresponding Media ID (value)
var languageToMediaMapping = {
"da": "DANISH_MEDIA_ID",
"en": "ENGLISH_MEDIA_ID",
"de": "GERMAN_MEDIA_ID"
};
// Read lang cookie.
var match = /(^|; )lang=([a-z]{2})($|;)/.exec(document.cookie);
if (match) {
var language = match[2];
var mediaId = languageToMediaMapping[language];
if (mediaId) {
window._urq = window._urq || [];
window._urq.push(["setMediaId", mediaId]);
}
}
}
initUserReport();
</script>
<script src="https://sak.userreport.com/your_company/launcher.js"
id="userreport-launcher-script" async></script>
In order to test visit your website with ?__urp=test_invite
parameter (e.g. http://example.com?__urp=test_invite
) to make sure that invitation is shown in correct language. If script is unable to determine media - it will notify show notification in this regards.
Example. Choosing media based on JavaScript variable
Your website have a lot of users and focused in following subjects:
- Cooking
- Relations
- Parenting
- Housing
Each section have a lot of visits, different editors are responsible for content. So you want to have this sections as Medias, so you can ask different questions on each section and individual satisfaction reports. If CMS you are using to run website allow adds page category to its address (e.g. all articles for Cooking are located under example.com/cooking) then you can use Advanced rules to assign media to each section.
However it is not always possible to do through URLs, then you need to set media ID in snippet. Most often CMS add some sort of meta object to the page that includes information about content, such as page category. For instance
<script>
window.PageData = {
"mainCategory": "cooking",
"labels": [ "pasta", "shrimps" ],
"author": "Luciano Markiso"
};
</script>
To detect media based on variable available in JavaScript object you need:
- Create three UserReport media for each website section you want to define as separate media, don't enter media detection rules
- Get ID of each created media
- Modify UserReport snippet so it gets page category from JavaScript object, chooses correct media and sets it to snippet
Based on example above, UserReport snippet can look like this.
<script>
function initUserReport() {
// Relation between website category (key) and corresponding Media ID (value)
var categoryToMediaMapping = {
"cooking": "COOKING_MEDIA_ID",
"relations": "RELATIONS_MEDIA_ID",
"parenting": "PARENTING_MEDIA_ID",
"housing": "HOUSING_MEDIA_ID"
};
var mainCategory = window.PageData.mainCategory;
var mediaId = categoryToMediaMapping[mainCategory];
if (mediaId) {
window._urq = window._urq || [];
window._urq.push(["setMediaId", mediaId]);
}
}
initUserReport();
</script>
<script src="https://sak.userreport.com/your_company/launcher.js"
id="userreport-launcher-script" async></script>
In order to test visit each section of your website with ?__urp=test_invite
parameter (e.g. http://example.com/cooking/?__urp=test_invite
) to make sure that invitation is shown in correct language. If script is unable to determine media - it will notify show notification in this regards.
Setting Section ID
Unlike media that must be distinct for pageview, pageview may be attributed by multiple sections. For instance, when user is reading article about football match, it belongs to Sports and Football categories. Once you have created you may not specify URL-based rules, obtain section ID and from meta tag shown on Advanced tab, like on example below
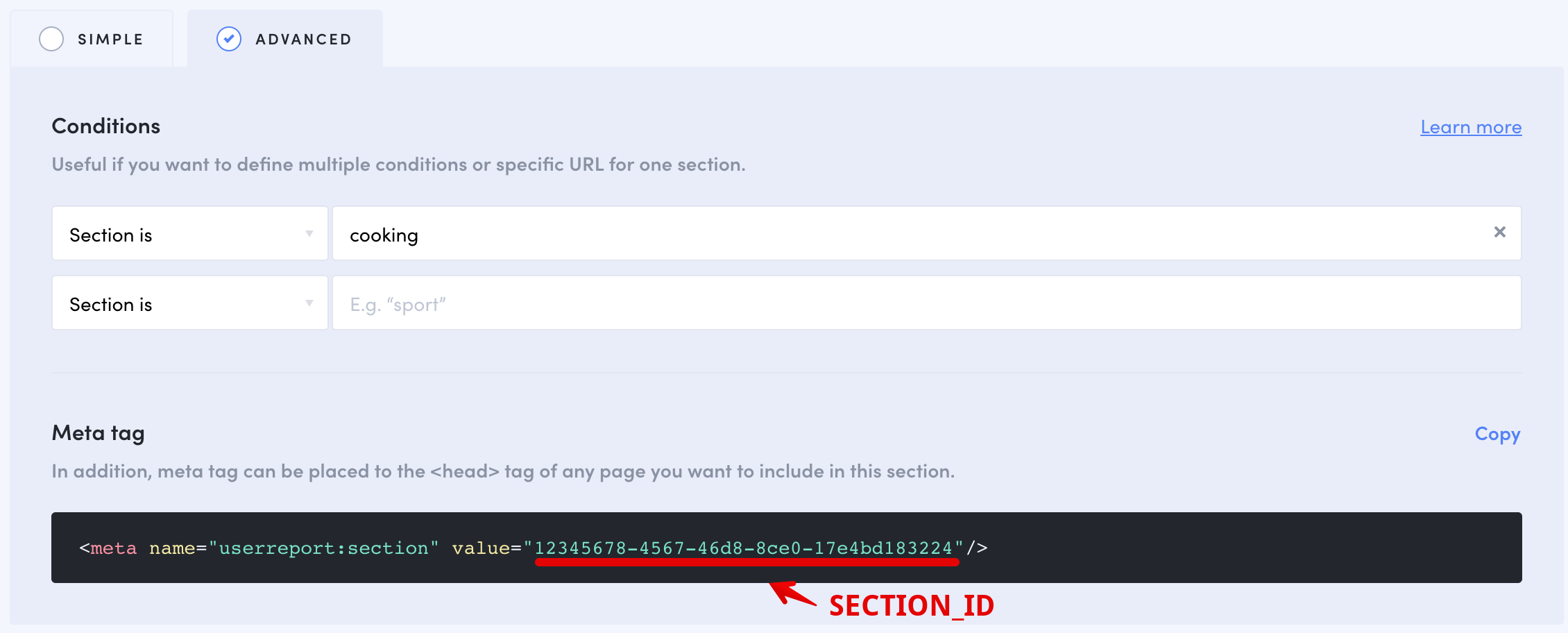
Having this value you can place snippet
<script>
window._urq = window._urq || [];
window._urq.push(["setSectionId", "12345678-4567-4af6-8f32-2a97ab508426"]);
</script>
<script src="https://sak.userreport.com/your_company/launcher.js"
id="userreport-launcher-script" async></script>
This will measure section for Kits regardless of page address where snippet is deployed.
Example. Choosing section based on JavaScript variables
You run medium size website with cooking recipes. You have categories for different cousins (European, Asian, American) and featured authors (Gordon Ramsay, Jamie Oliver, Giada De Laurentees, Guy Fieri). You want to get audience of each cuisine and featured author to showcase advertiser audience on these sections.
Most often, information about content is added to page by CMS
<script>
window.PageData = {
"cuisine": "american",
"labels": [ "burger", "big food" ],
"author": "Guy Fieri"
};
</script>
You need to modify snippet so it gets section ID for cuisine, section ID for author and pushes them to JavaScript (sections must be created before-hands).
Here is an example of implementation
<script>
function initUserReport() {
// Relation between website category (key) and corresponding Media ID (value)
var cuisineMapping = {
"american": "AMERICAN_SECTION_ID",
"asian": "ASIAN_SECTION_ID",
"european": "EUROPEAN_SECTION_ID"
};
var cuisineMapping = {
"Gordon Ramsay": "Gordon_Ramsay_SECTION_ID",
"Jamie Oliver": "Jamie_Oliver_SECTION_ID",
"Giada De Laurentees": "Giada_De_Laurentees_SECTION_ID",
"Guy Fieri": "Guy_Fieri_SECTION_ID"
};
window._urq = window._urq || [];
var cuisine = window.PageData.cuisine;
var cuisineSectionId = cuisineMapping[cuisine];
if (cuisineSectionId) {
window._urq.push(["setSectionId",cuisineSectionId]);
}
var author = window.PageData.author;
var authorSectionId = cuisineMapping[authorSectionId];
if (authorSectionId) {
window._urq.push(["setSectionId",authorSectionId]);
}
}
initUserReport();
</script>
<script src="https://sak.userreport.com/your_company/launcher.js"
id="userreport-launcher-script" async></script>